Abstract Syntax Tree Parsers¶
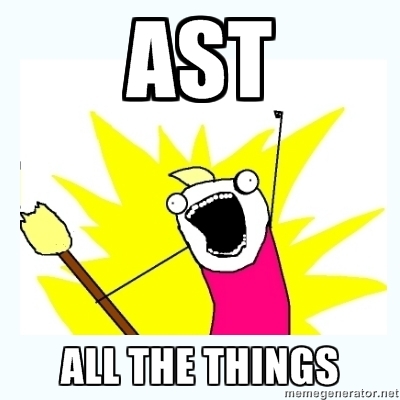
This module creates abstract syntax trees using external tools (GCC-XML, pycparser) of C/C++ code.
author: | Anthony Scopatz <scopatz@gmail.com> |
---|
AST Parsers API¶
-
class
xdress.astparsers.
ParserPlugin
[source]¶ This is a base plugin for tools that wish to wrap parsing. It should not be used directly.
The __init__() method may take no arguments or keyword arguments.
-
requires
= ('xdress.base',)¶ This plugin requires ‘xdress.base’.
-
-
xdress.astparsers.
clang_parse
(*args, **kwargs)[source]¶ Use clang to parse a file.
Parameters: filename : str
The path to the file.
includes: list of str, optional :
The list of extra include directories to search for header files.
defines: list of str, optional :
The list of extra macro definitions to apply.
undefines: list of str, optional :
The list of extra macro undefinitions to apply.
extra_parser_args : list of str, optional
Further command line arguments to pass to the parser.
verbose : bool, optional
Flag to display extra information while describing the class. Ignored.
debug : bool, optional
Flag to enable/disable debug mode. Currently ignored.
builddir : str, optional
Ignored. Exists only for compatibility with gccxml_describe.
language : str
Valid language flag.
clang_includes : list of str, optional
clang-specific includes paths.
Returns: tu : libclang TranslationUnit object
-
xdress.astparsers.
dumpast
(filename, parsers, sourcedir, includes=(), defines=('XDRESS', ), undefines=(), verbose=False, debug=False, builddir='build')[source]¶ Prints an abstract syntax tree to stdout.
-
xdress.astparsers.
gccxml_parse
(*args, **kwargs)[source]¶ Use GCC-XML to parse a file. This function is automatically memoized.
Parameters: filename : str
The path to the file.
includes : list of str, optional
The list of extra include directories to search for header files.
defines : list of str, optional
The list of extra macro definitions to apply.
undefines : list of str, optional
The list of extra macro undefinitions to apply.
extra_parser_args : list of str, optional
Further command line arguments to pass to the parser.
verbose : bool, optional
Flag to display extra information while describing the class.
debug : bool, optional
Flag to enable/disable debug mode.
builddir : str, optional
Location of – often temporary – build files.
clang_includes : ignored
Returns: root : XML etree
An in memory tree representing the parsed file.
-
xdress.astparsers.
pick_parser
(file_or_lang, parsers)[source]¶ Determines the parse to use for a file.
Parameters: file_or_lang : str
The path to the file OR a valid language flag.
parsers : str, list, or dict, optional
The parser / AST to use to use for the file. Currently ‘clang’, ‘gccxml’, and ‘pycparser’ are supported, though others may be implemented in the future. If this is a string, then this parser is used. If this is a list, this specifies the parser order to use based on availability. If this is a dictionary, it specifies the order to use parser based on language, i.e.
{'c' ['pycparser', 'gccxml'], 'c++': ['gccxml', 'pycparser']}
.Returns: parser : str
The name of the parser to use.
-
xdress.astparsers.
pycparser_parse
(*args, **kwargs)[source]¶ Use pycparser to parse a file. This functions is automatically memoized.
Parameters: filename : str
The path to the file.
includes : list of str, optional
The list of extra include directories to search for header files.
defines : list of str, optional
The list of extra macro definitions to apply.
undefines : list of str, optional
The list of extra macro undefinitions to apply.
extra_parser_args : list of str, optional
Further command line arguments to pass to the parser.
verbose : bool, optional
Flag to display extra information while describing the class.
debug : bool, optional
Flag to enable/disable debug mode.
builddir : str, optional
Location of – often temporary – build files.
clang_includes : ignored
Returns: root : AST
A pycparser abstract syntax tree.